rustc_errors: only box the `diagnostic` field in `DiagnosticBuilder`.
I happened to need to do the first change (replacing `allow_suggestions` with equivalent functionality on `Diagnostic` itself) as part of a larger change, and noticed that there's only two fields left in `DiagnosticBuilderInner`.
So with this PR, instead of a single pointer, `DiagnosticBuilder` is two pointers, which should work just as well for passing *it* by value (and may even work better wrt some operations, though probably not by much).
But anything that was already taking advantage of `DiagnosticBuilder` being a single pointer, and wrapping it further (e.g. `Result<T, DiagnosticBuilder>` w/ non-ZST `T`), ~~will probably see a slowdown~~, so I want to do a perf run before even trying to propose this.
Check that `#[rustc_must_implement_one_of]` is applied to a trait
`#[rustc_must_implement_one_of]` only makes sense when applied to a trait, so it's sensible to emit an error otherwise.
Store def_id_to_hir_id as variant in hir_owner.
If hir_owner is Owner(_), the LocalDefId is pointing to an owner, so the ItemLocalId is 0.
If the HIR node does not exist, we store Phantom.
Otherwise, we store the HirId associated to the LocalDefId.
Related to #89278
r? `@oli-obk`
Switch pretty printer to block-based indentation
This PR backports 401d60c042 from the `prettyplease` crate into `rustc_ast_pretty`.
A before and after:
```diff
- let res =
- ((::alloc::fmt::format as
- for<'r> fn(Arguments<'r>) -> String {format})(((::core::fmt::Arguments::new_v1
- as
- fn(&[&'static str], &[ArgumentV1]) -> Arguments {Arguments::new_v1})((&([("test"
- as
- &str)]
- as
- [&str; 1])
- as
- &[&str; 1]),
- (&([]
- as
- [ArgumentV1; 0])
- as
- &[ArgumentV1; 0]))
- as
- Arguments))
- as String);
+ let res =
+ ((::alloc::fmt::format as
+ for<'r> fn(Arguments<'r>) -> String {format})(((::core::fmt::Arguments::new_v1
+ as
+ fn(&[&'static str], &[ArgumentV1]) -> Arguments {Arguments::new_v1})((&([("test"
+ as &str)] as [&str; 1]) as
+ &[&str; 1]),
+ (&([] as [ArgumentV1; 0]) as &[ArgumentV1; 0])) as
+ Arguments)) as String);
```
Previously the pretty printer would compute indentation always relative to whatever column a block begins at, like this:
```rust
fn demo(arg1: usize,
arg2: usize);
```
This is never the thing to do in the dominant contemporary Rust style. Rustfmt's default and the style used by the vast majority of Rust codebases is block indentation:
```rust
fn demo(
arg1: usize,
arg2: usize,
);
```
where every indentation level is a multiple of 4 spaces and each level is indented relative to the indentation of the previous line, not the position that the block starts in.
By itself this PR doesn't get perfect formatting in all cases, but it is the smallest possible step in clearly the right direction. More backports from `prettyplease` to tune the ibox/cbox indent levels around various AST node types are upcoming.
The `print_expr` method already places an `ibox(INDENT_UNIT)` around
every expr that gets printed. Some exprs were then using `self.head`
inside of that, which does its own `cbox(INDENT_UNIT)`, resulting in two
levels of indentation:
while true {
stuff;
}
This commit fixes those cases to produce the expected single level of
indentation within every expression containing a block.
while true {
stuff;
}
Previously the pretty printer would compute indentation always relative
to whatever column a block begins at, like this:
fn demo(arg1: usize,
arg2: usize);
This is never the thing to do in the dominant contemporary Rust style.
Rustfmt's default and the style used by the vast majority of Rust
codebases is block indentation:
fn demo(
arg1: usize,
arg2: usize,
);
where every indentation level is a multiple of 4 spaces and each level
is indented relative to the indentation of the previous line, not the
position that the block starts in.
Create `core::fmt::ArgumentV1` with generics instead of fn pointer
Split from (and prerequisite of) #90488, as this seems to have perf implication.
`@rustbot` label: +T-libs
Render more readable macro matcher tokens in rustdoc
Follow-up to #92334.
This PR lifts some of the token rendering logic from https://github.com/dtolnay/prettyplease into rustdoc so that even the matchers for which a source code snippet is not available (because they are macro-generated, or any other reason) follow some baseline good assumptions about where the tokens in the macro matcher are appropriate to space.
The below screenshots show an example of the difference using one of the gnarliest macros I could find. Some things to notice:
- In the **before**, notice how a couple places break in between `$(....)`↵`*`, which is just about the worst possible place that it could break.
- In the **before**, the lines that wrapped are weirdly indented by 1 space of indentation relative to column 0. In the **after**, we use the typical way of block indenting in Rust syntax which is put the open/close delimiters on their own line and indent their contents by 4 spaces relative to the previous line (so 8 spaces relative to column 0, because the matcher itself is indented by 4 relative to the `macro_rules` header).
- In the **after**, macro_rules metavariables like `$tokens:tt` are kept together, which is how just about everybody writing Rust today writes them.
## Before
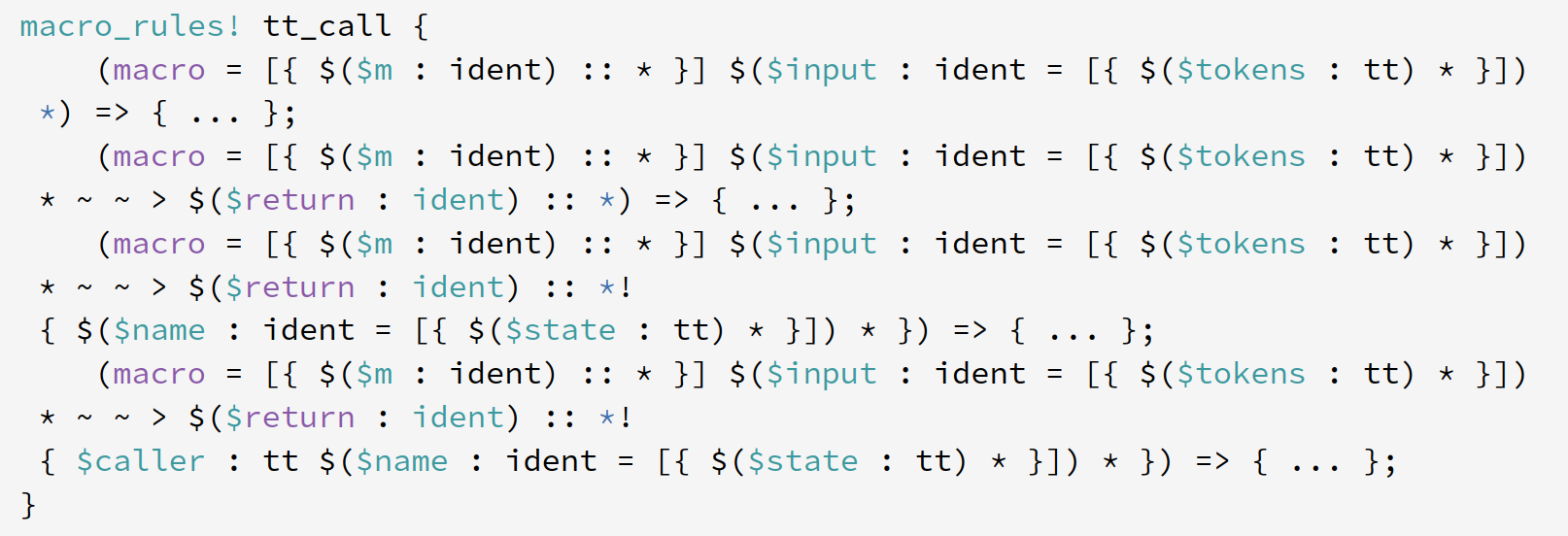
## After
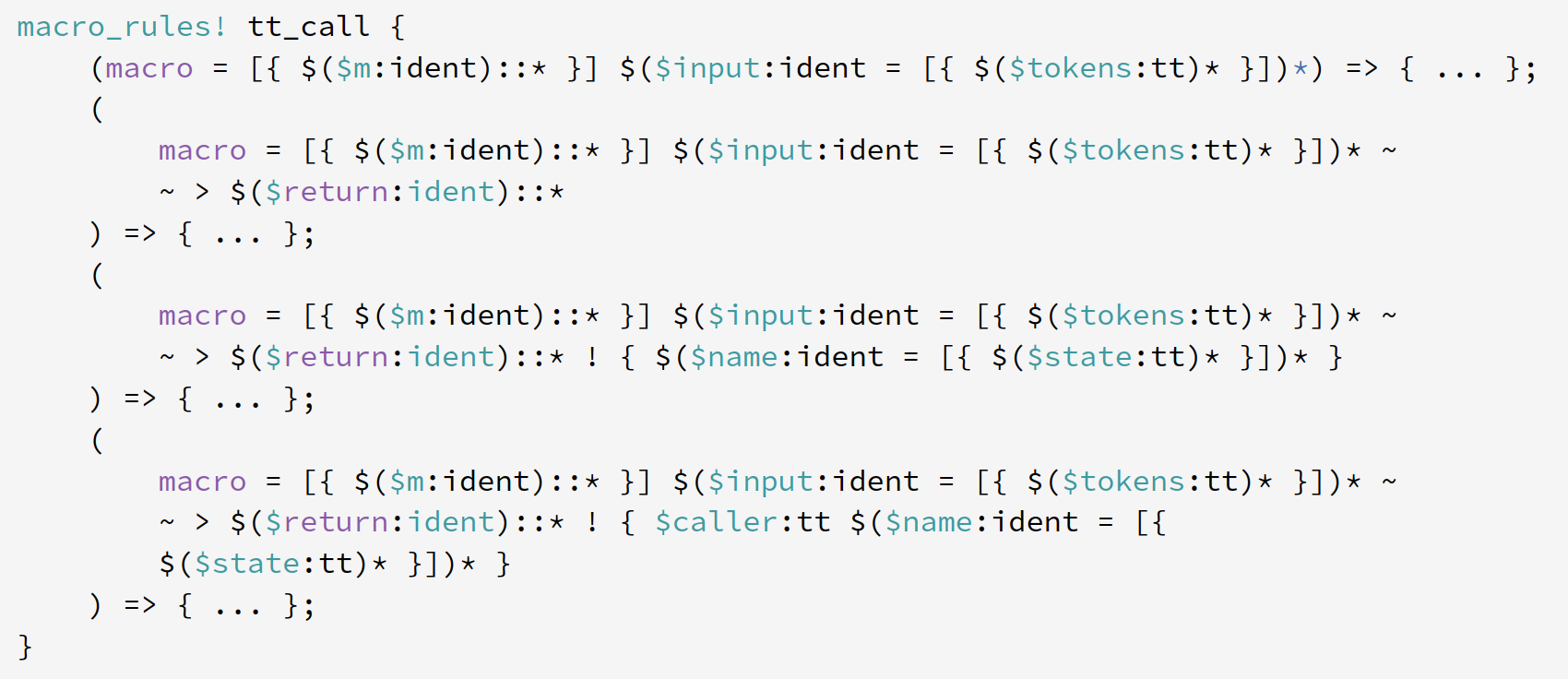
r? `@camelid`
Rename _args -> args in format_args expansion
As observed in https://github.com/rust-lang/rust/pull/91359#discussion_r786058960, prior to that PR this variable was sometimes never used, such as in the case of:
```rust
println!("");
// used to expand to:
::std::io::_print(
::core::fmt::Arguments::new_v1(
&["\n"],
&match () {
_args => [],
},
),
);
```
so the leading underscore in `_args` was used to suppress an unused variable lint. However after #91359 the variable is always used when present, as the unused case would instead expand to:
```rust
::std::io::_print(::core::fmt::Arguments::new_v1(&["\n"], &[]));
```
Add note suggesting that predicate may be satisfied, but is not `const`
Not sure if we should be printing this in addition to, or perhaps _instead_ of the help message:
```
help: the trait `~const Add` is not implemented for `NonConstAdd`
```
Also added `ParamEnv::is_const` and `PolyTraitPredicate::is_const_if_const` and, in a separate commit, used those in other places instead of `== hir::Constness::Const`, etc.
r? ````@fee1-dead````
remove unused `jemallocator` crate
When it was noticed that the rustc binary wasn't actually using jemalloc via `#[global_allocator]` and that was removed, the dependency remained.
Tests pass locally with a `jemalloc = true` build, but I'll trigger a try build to ensure I haven't missed an edge-case somewhere.
r? ```@ghost``` until that completes
Add `intrinsics::const_deallocate`
Tracking issue: #79597
Related: #91884
This allows deallocation of a memory allocated by `intrinsics::const_allocate`. At the moment, this can be only used to reduce memory usage, but in the future this may be useful to detect memory leaks (If an allocated memory remains after evaluation, raise an error...?).
Rollup of 10 pull requests
Successful merges:
- #92611 (Add links to the reference and rust by example for asm! docs and lints)
- #93158 (wasi: implement `sock_accept` and enable networking)
- #93239 (Add os::unix::net::SocketAddr::from_path)
- #93261 (Some unwinding related cg_ssa cleanups)
- #93295 (Avoid double panics when using `TempDir` in tests)
- #93353 (Unimpl {Add,Sub,Mul,Div,Rem,BitXor,BitOr,BitAnd}<$t> for Saturating<$t>)
- #93356 (Edit docs introduction for `std::cmp::PartialOrd`)
- #93375 (fix typo `documenation`)
- #93399 (rustbuild: Fix compiletest warning when building outside of root.)
- #93404 (Fix a typo from #92899)
Failed merges:
r? `@ghost`
`@rustbot` modify labels: rollup
Add links to the reference and rust by example for asm! docs and lints
These were previously removed in #91728 due to broken links.
cc ``@ehuss`` since this updates the rust-by-example submodule
Fix debuginfo for pointers/references to unsized types
This PR makes the compiler emit fat pointer debuginfo in all cases. Before, we sometimes got thin-pointer debuginfo, making it impossible to fully interpret the pointed to memory in debuggers. The code is actually cleaner now, especially around generation of trait object pointer debuginfo.
Fixes https://github.com/rust-lang/rust/issues/92718
~~Blocked on https://github.com/rust-lang/rust/pull/92729.~~
Suggest tuple-parentheses for enum variants
This follows on from #86493 / #86481, making the parentheses suggestion. To summarise, given the following code:
```rust
fn f() -> Option<(i32, i8)> {
Some(1, 2)
}
```
The current output is:
```
error[E0061]: this enum variant takes 1 argument but 2 arguments were supplied
--> b.rs:2:5
|
2 | Some(1, 2)
| ^^^^ - - supplied 2 arguments
| |
| expected 1 argument
error: aborting due to previous error
For more information about this error, try `rustc --explain E0061`.
```
With this change, `rustc` will now suggest parentheses when:
- The callee is expecting a single tuple argument
- The number of arguments passed matches the element count in the above tuple
- The arguments' types match the tuple's fields
```
error[E0061]: this enum variant takes 1 argument but 2 arguments were supplied
--> b.rs:2:5
|
2 | Some(1, 2)
| ^^^^ - - supplied 2 arguments
|
help: use parentheses to construct a tuple
|
2 | Some((1, 2))
| + +
```
LLDB does not seem to see fields if they are marked with DW_AT_artificial
which breaks pretty printers that use these fields for decoding fat pointers.
Only traverse attrs once while checking for coherence override attributes
In coherence, while checking for negative impls override attributes: only traverse the `DefId`s' attributes once.
This PR is an easy way to get back some of the small perf loss in #93175